链表
1. 链表定义
1 2 3 4 5
| type Student struct { Name string Next* Student }
|
定义一个简单的链表
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| package main
import "fmt"
type Student struct { Name string Age int Score float32 next* Student }
func main() { var head Student head.Name = "hua" head.Age = 18 head.Score = 100
var stu1 Student stu1.Name = "stu1" stu1.Age = 20 stu1.Score = 20
head.next = &stu1
var p *Student = &head for p != nil { fmt.Println(*p) p = p.next } }
|
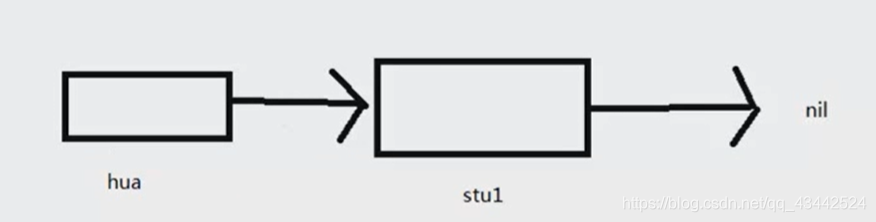
输出:
1 2
| {hua 18 100 0xc000054330} {stu1 20 20 <nil>}
|
2. 尾部插入法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| package main
import ( "fmt" "math/rand" )
type Student struct { Name string Age int Score float32 next* Student }
func trans(p *Student) { for p!=nil{ fmt.Println(*p) p = p.next } }
func main() {
var head Student head.Name = "hua" head.Age = 18 head.Score = 100
var tail = &head for i := 0; i < 10; i++ { var stu = Student{ Name: fmt.Sprintf("stu%d", i), Age: rand.Intn(100), Score: rand.Float32()*100, } tail.next = &stu tail = &stu } trans(&head) }
|
输出:
1 2 3 4 5 6 7 8 9 10 11
| {hua 18 100 0xc0000a6360} {stu0 81 94.05091 0xc0000a6390} {stu1 47 43.77142 0xc0000a63c0} {stu2 81 68.682304 0xc0000a63f0} {stu3 25 15.651925 0xc0000a6420} {stu4 56 30.091187 0xc0000a6450} {stu5 94 81.36399 0xc0000a6480} {stu6 62 38.06572 0xc0000a64b0} {stu7 28 46.888985 0xc0000a64e0} {stu8 11 29.310184 0xc0000a6510} {stu9 37 21.855305 <nil>}
|
3. 头部插入法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| package main
import ( "fmt" "math/rand" )
type Student struct { Name string Age int Score float32 next* Student }
func trans(p *Student) { for p!=nil{ fmt.Println(*p) p = p.next } }
func main() {
var head *Student = new(Student) head.Name = "hua" head.Age = 18 head.Score = 100
for i := 0; i < 10; i++ { stu := Student{ Name: fmt.Sprintf("stu%d", i), Age: rand.Intn(100), Score: rand.Float32()*100, } stu.next = head head = &stu } trans(head) }
|
输出:
1 2 3 4 5 6 7 8 9 10 11
| {stu9 37 21.855305 0xc000054510} {stu8 11 29.310184 0xc0000544e0} {stu7 28 46.888985 0xc0000544b0} {stu6 62 38.06572 0xc000054480} {stu5 94 81.36399 0xc000054450} {stu4 56 30.091187 0xc000054420} {stu3 25 15.651925 0xc0000543f0} {stu2 81 68.682304 0xc0000543c0} {stu1 47 43.77142 0xc000054390} {stu0 81 94.05091 0xc000054360} {hua 18 100 <nil>}
|